Building a Chatbot with React, Express, and Google’s Gemini AI
March 3, 2024
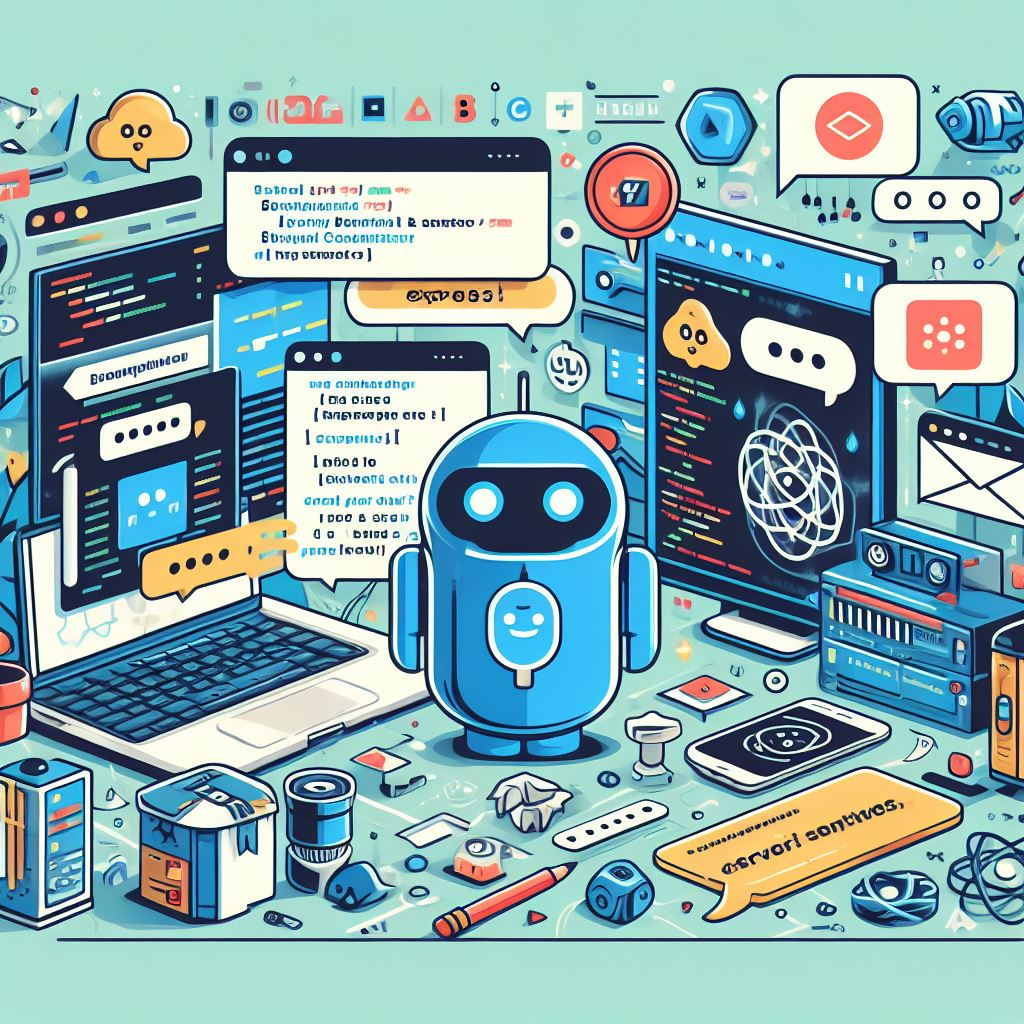
Abstract
This tutorial provides a detailed guide for constructing a sophisticated chatbot using modern web development technologies. By combining React for the frontend, Express for the backend, and Google’s Gemini AI for intelligent responses, developers can create an interactive conversational experience. The guide covers everything from setting up the development environment to integrating frontend and backend functionalities, resulting in the deployment of a fully functional chatbot application.
Step-by-Step Guide
1. Set Up Environment
- Create a new React project using Create React App.
- Navigate to the project directory and install necessary dependencies.
@google/generative-ai: "⁰.2.1" (Google generative AI service)
concurrently: "⁸.2.2" (Runs multiple commands concurrently)
cors: "².8.5" (Enables Cross-Origin Resource Sharing)
dotenv: "¹⁶.4.5" (Loads environment variables from a .env file)
express: "⁴.18.3" (Web framework for Node.js)
nodemon: "³.1.0" (Monitors changes in Node.js files and restarts the server)
prismjs: "¹.29.0" (Provides syntax highlighting for code blocks)
react-markdown: "⁹.0.1" (Renders Markdown content in React applications)
2. Obtain Gemini API Key
- Visit the Gemini documentation website and sign in with your Google account. `https://ai.google.dev`
- Follow the instructions to obtain your Gemini API key.
3. Initialize Frontend
- Create a react project using create react app.
npx create-react-app chatbot-app
- Clean up the default files generated by Create React App (e.g., App.js, App.css).
- Create a new index.css file to style the basic layout of the chat app.
4. Create Environment Variables
- Create a .env file in the project root and store your Gemini API key as GOOGLE_GEN_AI_KEY=<your_api_key>.
5. Build Chat Interface
- Design the chat interface with appropriate HTML elements (e.g., div, p, textarea, button).
- Use conditional rendering to display appropriate buttons based on the presence of errors.
- Implement state management using React hooks (useState) to handle user input and error messages.
6. Style Chat Interface
- Style the chat interface using CSS to improve its appearance and usability.
- Apply styling to elements such as buttons, input fields, error messages, and chat history.
7. Set Up Backend
- Create a new file named server.js to set up the backend server.
- Install nodemon package to automatically restart the server on file changes.
- Require necessary packages like Express, Cors, dotenv, and Google Generative AI.
8. Link Frontend and Backend
- Configure scripts in package.json to run both frontend and backend servers concurrently.
- Use concurrently package to manage multiple scripts.
9. Define Endpoint and Handle Requests
- Define a POST endpoint (e.g., /gemini) to receive requests from the frontend.
- Use Express to handle incoming requests.
- Extract the request body to get the chat history and user message.
- Utilize Google Generative AI to generate a response based on the provided chat history and message.
- Send the generated response back to the frontend.
10. Frontend Integration
- Create a function (e.g., getResponse) in your frontend to handle sending requests to the backend.
- Use Fetch API or any other method to send a POST request to the defined endpoint on your backend.
- Include the chat history and user message in the request body.
- Receive and handle the response from the backend.
- Update the UI to display the received response in the chat interface.
11. Implement Chat Interface
- Design and create a chat interface using HTML, CSS, and JavaScript.
- Include input field(s) for users to type their messages.
- Display previous messages and responses in the chat window.
- Implement functionality to send messages to the backend and receive responses asynchronously.
- Allow users to clear the chat history and input field(s).
In this point your code should look like
https://github.com/LaXnZ/react-gemini-chatbot/tree/3831643aeed21b9e5e251735fecce1b1021fc3a2
12. Testing and Debugging
- Test the chatbot thoroughly, including sending various types of messages to see how it responds.
- Debug any issues that arise during testing, both in the frontend and backend code.
- Ensure that the chatbot handles edge cases gracefully and provides helpful error messages when needed.
Conclusion
This comprehensive guide empowers developers to build a sophisticated chatbot application using React, Express, and Gemini. By following the outlined steps and best practices, developers can create immersive conversational experiences that cater to diverse user interactions, paving the way for innovative web-based communication solutions.
Thank you for reading my article! If you enjoyed it and would like to support my work, please consider making a donation. Unfortunately, I’m not in an eligible country to receive payouts from Medium, so your support would mean a lot to me.
You can donate through the following link: Donate Here
Thank you for your generosity!
My personal website: laxnz.me